Things we need
1. vJoy Software
2. Arduino IDE
3. Arduino Nano
4. 2 Joystick (any)
Here is the wiring diagram
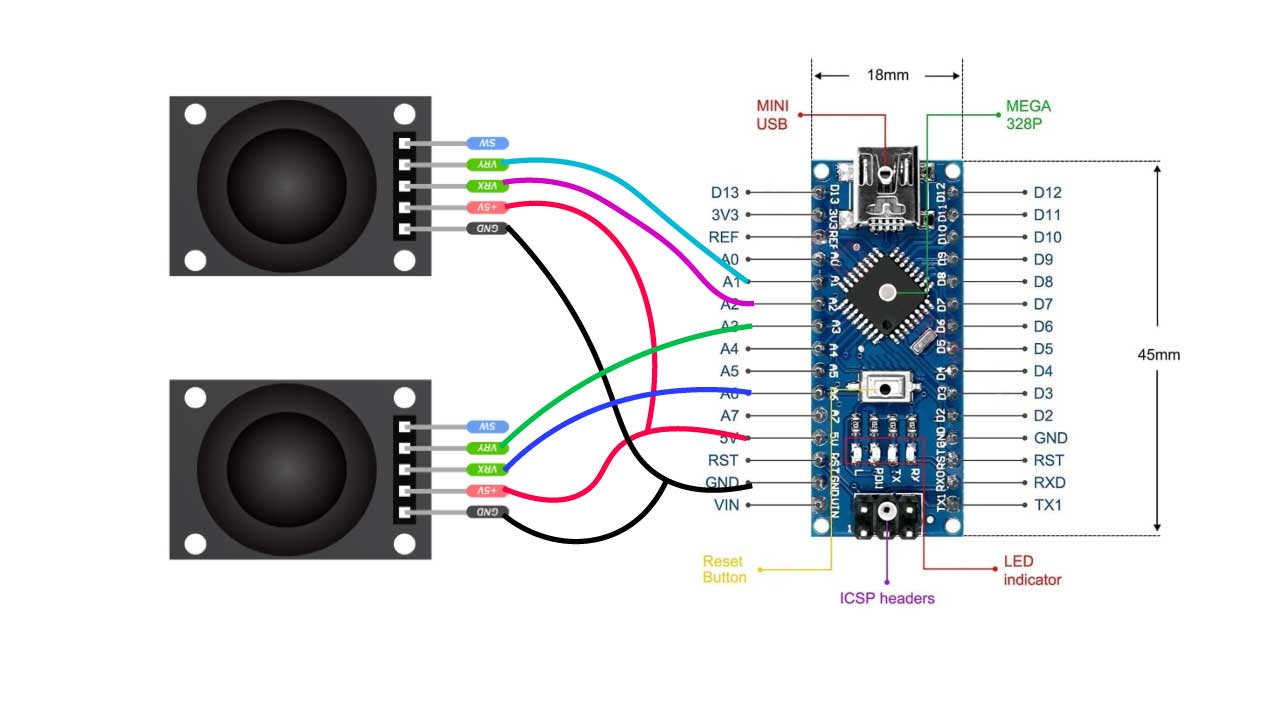
Code for Computer Side (PYTHON) Ex-mycode.py
import serial
import pyvjoy
# Connect to Arduino
ser = serial.Serial('COM4', 115200)
joystick = pyvjoy.VJoyDevice(1)
print("Serial to vJoy is running...")
while True:
data = ser.readline().decode('utf-8', errors='ignore').strip()
if data.startswith('T:'):
try:
parts = data.split()
throttle = int(parts[0][2:])
yaw = int(parts[1][2:])
roll = int(parts[2][2:])
pitch = int(parts[3][2:])
# Send values to vJoy
joystick.set_axis(pyvjoy.HID_USAGE_X, roll * 32)
joystick.set_axis(pyvjoy.HID_USAGE_Y, pitch * 32)
joystick.set_axis(pyvjoy.HID_USAGE_Z, yaw * 32)
joystick.set_axis(pyvjoy.HID_USAGE_SL0, throttle * 32)
except Exception as e:
print("Error:", e)
Code for Arduino Nano (C/C++/Arduino) Ex- Nano.ino
// Define analog pin connections
#define THROTTLE_PIN A6
#define YAW_PIN A3
#define ROLL_PIN A1
#define PITCH_PIN A2
void setup() {
Serial.begin(115200);
}
void loop() {
// Read analog values
int throttleValue = analogRead(THROTTLE_PIN);
int yawValue = analogRead(YAW_PIN);
int rollValue = analogRead(ROLL_PIN);
int pitchValue = analogRead(PITCH_PIN);
// Send joystick values via serial
Serial.print("T:"); Serial.print(throttleValue);
Serial.print(" Y:"); Serial.print(yawValue);
Serial.print(" R:"); Serial.print(rollValue);
Serial.print(" P:"); Serial.println(pitchValue);
// Small delay for stability
delay(10);
}